Today we are going to learn how to upload the file or image in react. Image upload or file upload is a common requirement of the application.
Next Couldn't find a `pages` directory. Please create one under the project root. This is because Next uses the pages directory and the files in them to map its routes. This means if we have a file called index.js in our pages folder, Next would try to use the component in it as our entry point. Let’s create the pages folder and the index. Npx create-react-app my-app. Where my-app is the name of the folder for your application. This may take a few minutes to create the React application and install its dependencies. Note: If you've previously installed create-react-app globally via npm install -g create-react-app, we recommend you uninstall the package using npm uninstall -g. Create React App. Create React App is a comfortable environment for learning React, and is the best way to start building a new single-page application in React. It sets up your development environment so that you can use the latest JavaScript features, provides a nice developer experience, and optimizes your app for production.
Before start learning react image or file upload. We need a `React Project`. If you don’t know how to create a new project. Follow this tutorial.
React And Rctimagedownloader Folders Created Under Documents Pdf
So, Let’s get started react image or file upload
Create template
To upload file we need a html template. In this template we will create `file input` element that allows to us to choose the file and a button to upload file. To create it open the `App.js` file and update the render function with the below code.
You may notic that we have two method in this `HTML` Fist `fileChangedHandler` and second `submit`
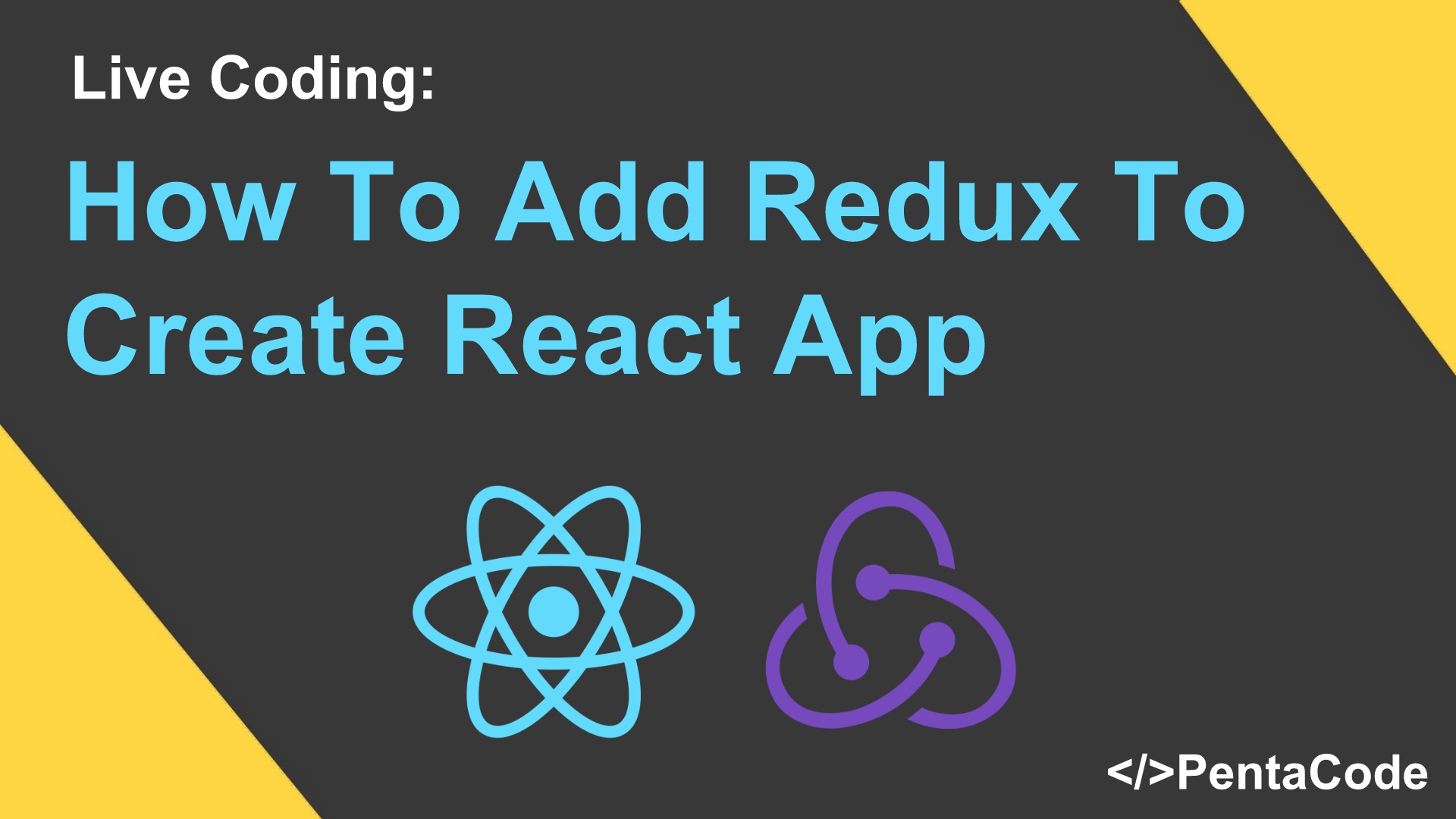
Let’s write `fileChangedHandler` function
The `fileChangedHandler` method will called when the user choose file. It will get the file object of selected file and store in the `selectedFile` state.
Now we will write another function which is responsible to upload the file into the server.
In this function first we create a new object of the `FormData` and then append the file. then send the request to the server
Here we have crated a `formData` object. We will store the file object to the `formData` and then upload it to the server.
After the all changes our file will looks like this

Running application:
Run the application using npm run start
Preview
Our application will looks like this:
If you loved this tutorial, Please share and if you have any question please comment
There are multiple ways to upload a file using React. I’m going to explain the steps to upload a single file, multiple files, and files and data in React.
We’re going to cover every scenario you might come across to upload a file from inside of a React component. These are:
How to Upload a File in React
We’ll start with the easiest and most common scenario, which is how to upload a single file to a server from a React component.
If you want to learn how to create a drag-and-drop file upload component in React, check out React Dropzone and File Upload in React.
Any type of file upload in React, or any front-end JavaScript library for that matter, requires an HTTP library to send the file data to a server.
I’m using the Fetch HTTP library in the following examples, but you can easily adapt them to work with other HTTP libraries like Axios or SuperAgent.
The example above uses a function, uploadFile, which takes a file object and passes that object to a POST request.
There are two things you need to remember when POSTing a file object:
- Never set the Context-Type Header.
- Pass the whole file object (Blob) to the body of the request.
Content-Type and Uploading a File
If you’ve made API requests in the past, then you probably used ‘application/
You do not need to set the content-type header when sending a file in a POST request.
React And Rctimagedownloader Folders Created Under Documents Electronically
File objects (Blobs) are not JSON, and therefore using an incorrect content-type will cause the request to fail. Web browsers automatically set the content-type header when sending a file in a POST request.
How to Upload Multiple Files in React using FormData
When we need to upload multiple files using Fetch, we have to use a new type of object called FormData.
FormData allows us to append multiple key/value pairs onto the object. After we’re done appending, we then pass it to the POST request’s body.
Let’s see an example of this below:
How to Upload Multiple Files and Other Data in React
There may be times when you need to send files and other data in a POST request. This can sometimes be referred to as multipart data.
For example, you may have a form which asks for a user’s first and last name, as well as a profile picture for their account avatar.
In this case, you’d want to use FormData again to send the file as well as the first name and last name. Let’s see how we’d use FormData to help us send all of this data together:
We’re simply using the formData.append method to create another key named ‘user’, and then passing the user object into the value of that key.
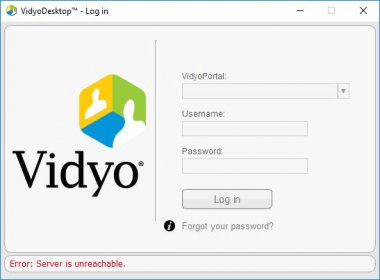